7 REST API Best Practices for Designing Robust APIs
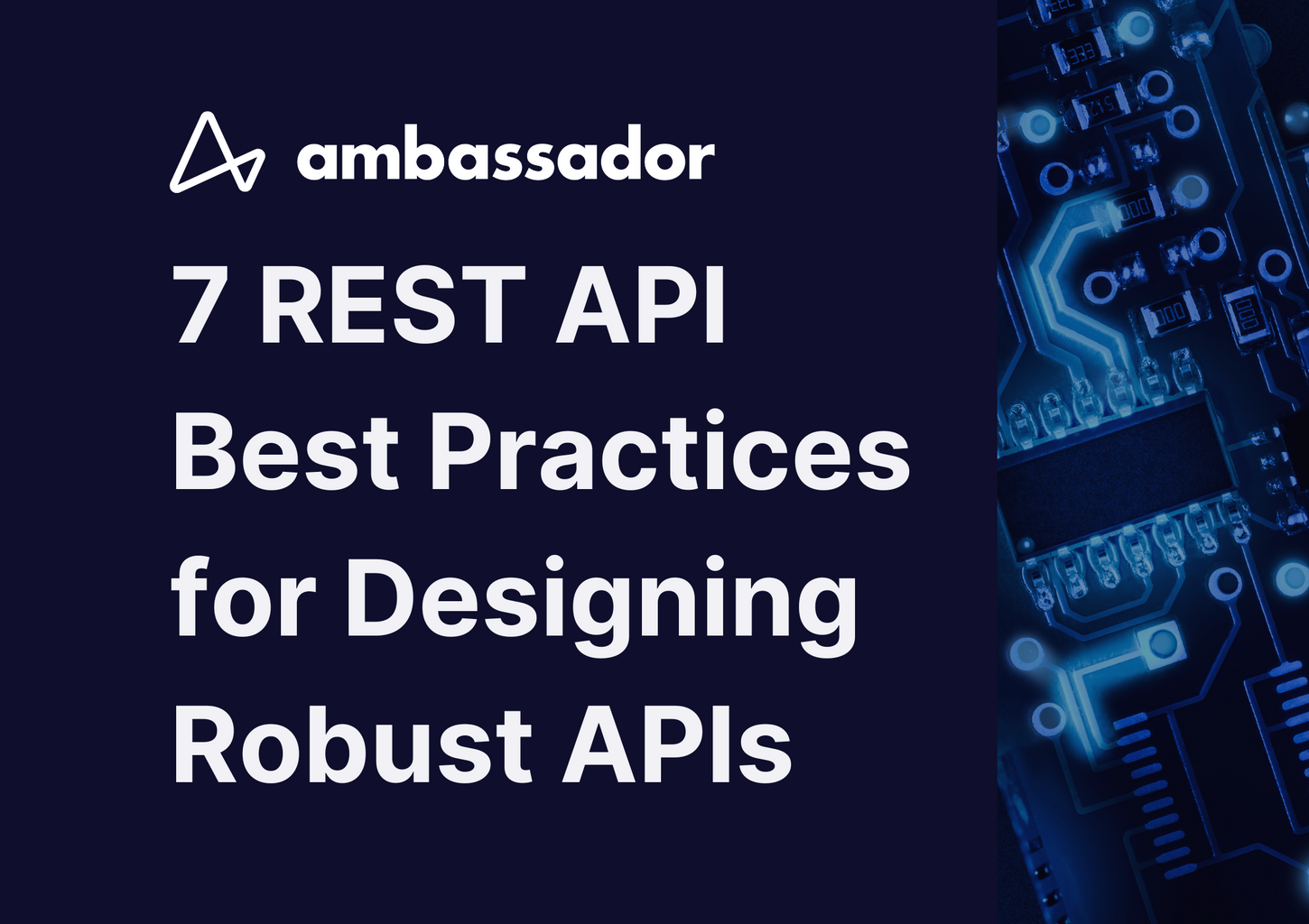
1. Utilize the Recommended Endpoint Naming Conventions
2. Use the Appropriate HTTP Method
3. Manage REST API Requests and Responses Effectively
4. Use the Appropriate Request Headers for Authentication
5. Know When to use Parameters vs. Query Parameters
6. Provide Informative and Actionable Error Messages
7. Create an API Documentation and Version your REST APIs
6. Adopt These Performance Optimization Techniques
7. Apply These Security Best Practices
Let’s Get RESTful
REST which stands for Representational State Transfer is an architectural style that governs how APIs are designed and built. REST’s popularity and ease of implementation make it the most preferred API architectural style for modern-day software development as compared to other protocols such as SOAP (simple object access protocol).
REST APIs or RESTful web services have become the backbone for efficient communication between client and server in modern-day software development. However, to build efficient and robust REST APIs, it is crucial to follow some standard best practices.
In this blog, we’ll explore REST API best practices that can help you build scalable, maintainable, secure, and robust APIs.
1. Utilize the Recommended Endpoint Naming Conventions
When you’re naming your HTTP request endpoints; it’s recommended that you follow naming conventions that are clear, precise, and aligned with the functionality they represent. If you are developing a RESTful web service for managing books in a library, you’d typically need to provide endpoints to retrieve a list of books, create a new book, update an existing book, and delete a book.
For almost all the functionalities, you should follow the standard naming convention pattern of /api/books. By using the /books path in the endpoint, we convey that this endpoint is responsible for managing books. For endpoints that update an existing book or delete it from the library, you can use the pattern /api/books/{id}.
2. Use the Appropriate HTTP Method
After you’ve named an endpoint, determine which HTTP methods to use based on the nature of the operation being performed. For context:
- GET requests are used to retrieve resources.
- PUT requests are typically employed for resource creation or replacement.
- POST requests are suitable for resource creation when the server assigns a unique identifier.
- PATCH requests allow for partial resource updates.
- DELETE requests, as the name implies, deletes the specified resource.
Going back to our previous example, the endpoint /api/books can be used for both creating a book or retrieving a list of books. So, what distinguishes the two? Here's how we can apply clear and precise naming conventions along with the correct HTTP methods to these endpoints:
- Retrieving a list of books: GET /api/books
- Creating a new book: POST /api/books
- Updating an existing book: PUT /api/books/{id}
- Deleting a book: DELETE /api/books/{id}
Here’s an example in Node.js to demonstrate the guidelines shared above:
app.get('/api/books', (req, res) => {// Retrieve and return a list of books});app.post('/api/books', (req, res) => {// Create a new book});app.put('/api/books/:id', (req, res) => {// Update the book with the specified ID});app.delete('/api/books/:id', (req, res) => {// Delete the book with the specified ID});
Following these naming conventions ensures consistency, promotes good API design, improves developer experience, and helps prevent confusion or errors when working with the API. It allows developers or API consumers to quickly grasp the purpose and functionality of each endpoint, facilitating efficient API consumption and development.
3. Manage REST API Requests and Responses Effectively
Once you’ve designed your endpoints, you need to manage your API requests and responses effectively. This will ensure a smooth and secure user experience and efficient communication between client and server.
For instance, you can use appropriate status codes in your responses so your client can handle them accordingly. Use 200 for all successful requests, 400 for client-based errors, and 500 for server-side errors. You should also regularly monitor your API’s usage and activities. This can be extremely helpful in identifying common errors and issues and any downtimes associated with your API. Ensure that you handle network timeouts as well. Set reasonable timeout limits and provide useful feedback to the client when such timeouts occur alongside the cause of these timeouts.
4. Use the Appropriate Request Headers for Authentication
Request headers to provide a way to pass authentication information from the client to the server. By utilizing appropriate request headers, you can implement authentication mechanisms like API keys, JWT (JSON Web Tokens), OAuth, or other custom authentication schemes. Here are some recommended request headers to use:
Authorization header: The Authorization header allows the client to include authentication credentials, such as tokens or API keys, in the request header. Here’s an example that uses the Bearer scheme to send the JWT after the scheme as authentication credentials.
Authorization: Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiIxMjM0NTY3ODkwIiwibmFtZSI6IkpvaG4gRG9lIiwiaWF0IjoxNTE2MjM5MDIyfQ.SflKxwRJSMeKKF2QT4fwpMeJf36POk6yJV_adQssw5c
- API key header: Another approach is using an x-api-key header to authenticate API requests. The client includes an API key, a unique identifier, in this header. The header carries the API key value, which is sent to the client for authentication.
x-api-key: ABCDEFG123456789
- Custom headers: Depending on the authentication mechanism implemented, custom headers can pass authentication-related information.
X-Auth-Token: abcd1234
5. Know When to use Parameters vs. Query Parameters
Web APIs often require you to work with additional information passed in the endpoint from the client. Understanding when to use path parameters (e.g., /api/books/{id}) versus query parameters (e.g., /api/books?category=fiction) is essential, especially for API consumers.
Path parameters are typically used to identify or retrieve a specific resource. Query parameters are more suitable for sorting the request data. You can also use it for filtering and pagination.
Returning to our previous example of books API, we’ve used path parameters for deleting a book or updating a book information using the endpoint /api/books/{id}. Here, the path parameter is id, and its value is the unique book identifier. On the other hand, let’s say you wish to retrieve all the books that belong to a certain category. You can use query parameters to specify this filter, such as /api/books?category=fiction. Here, the query parameter is category, and its value is fiction.
6. Provide Informative and Actionable Error Messages
A good API design ensures that your RESTful web services throw the correct error when needed. Having a robust error-handling mechanism aims to provide informative and actionable error messages.
You should wrap your code in Try-Catch blocks and return appropriate HTTP status codes, error payloads, and error messages that can be directly displayed to your users.
Consider the following API, which retrieves book information based on the path parameter id:
// Express.js exampleapp.get('/api/books/:id', (req, res) => {try {const bookId = req.params.id;// Retrieve book from the databaseconst book = database.getBookById(bookId);// Check if the book existsif (!book) {// Return a 404 Not Found errorres.status(404).json({error: 'Book not found',});return;}// Return the book detailsres.json(book);} catch (error) {// Handle any unexpected errorsconsole.error('Error occurred:', error);// Return a generic 500 Internal Server Error with a meaningful messageres.status(500).json({error: 'An unexpected error occurred',});}});
In the code snippet above, a 404 status is returned if the book doesn’t exist in the database. It also returns a 500 status with a generic error message if the API fails due to some other reason.
7. Create an API Documentation and Version your REST APIs
To increase the adoption and ease of use of your APIs, it’s crucial to create and maintain comprehensive API documentation. This documentation should provide information about available endpoints, request and response formats, authentication patterns, etc. OpenAPI, formerly known as Swagger, is a widely adopted specification for documenting REST APIs.
Along with documentation, you should also version your APIs. API versioning helps to easily manage changes and updates to an API while still maintaining compatibility with other versions of the APIs for clients. To version your APIs, you can assign unique identifiers or labels. Here are some common approaches to versioning your API:
- URL versioning: In this approach, the API version is included in the URL. For example, /api/v1/books indicate that this is version 1 of the API.
- Query parameter versioning: The version number is specified as a query parameter in the API request. For example, /api/books?version=1.
- Header versioning: The version number is a custom header in the API request. For example, Accept-Version: 1.
- Content negotiation versioning: The version is negotiated based on the Accept header or the media type of the request payload.
Versioning enables you to provide backward compatibility to your clients, facilitate the gradual adoption of changes for developers, and ensure stability throughout your various versions of APIs.
Edge Stack offers features for API documentation and version management, simplifying the process for developers and API consumers. You should check it out.
6. Adopt These Performance Optimization Techniques
Performance is an essential factor in determining the end-user experience of your APIs.
Let’s look at some common performance optimization techniques that you can adopt to create high-performing REST APIs:
- Use caching mechanisms to store frequently accessed data and reduce the load on the server. This can significantly improve response times when sending data between client and server and also reduce network traffic.
- Implement pagination to retrieve large datasets in smaller and more manageable chunks to ensure an optimized API design. By returning a limited number of results per page and providing navigation links, APIs can efficiently handle large amounts of data.
- Apply compression techniques, such as gzip, to reduce the size of data transferred between the client and server. This will improve response times for bandwidth-constrained environments and your application specific architectural constraints.
- Leverage rate limiting and throttling mechanisms to control the number of requests allowed from a particular client within a specific timeframe. This will prevent abuse and ensure fair usage of your REST API resources.
7. Apply These Security Best Practices
Security is a critical aspect of developing any software. However, security is also a tricky subject. By addressing some common vulnerabilities and implementing robust security measures, you can protect your APIs and the sensitive data they handle.
Here are some security best practices that you should add to your REST APIs against some common vulnerabilities:
- Properly validate and sanitize user inputs received on the server side. Also, encode the API responses to prevent malicious code execution. Following this will protect your REST APIs against vulnerabilities like SQL injection and cross-site scripting (XSS).
- Implement foolproof authentication and RBAC (Role-Based Access Control) mechanisms to protect your database resources from being accessed by unauthorized users. Your REST APIs are a gateway to your database, and you should ensure that all of your data is only accessed by users who are allowed to access it.
- Employ tools like Edge Stack as an API gateway solution for additional security features. It acts as a centralized entry point for all API traffic, enabling traffic encryption through SSL/TLS and offering protection against common attacks like DDoS (Distributed Denial-of-Service).
Let’s Get RESTful
Designing and developing REST APIs that adhere to best practices is essential for creating robust, scalable, and secure software systems. Now, you’ve seen how you can create robust, secure, and high-performing APIs right from design to development.
We also explored the role of Edge Stack as an API Gateway solution that facilitates the implementation of these best practices. Its comprehensive features, including SSO, WAF, version management, security enhancements, and traffic encryption, make it an ideal choice for a robust and secure API Gateway solution. Try it now or Schedule a Demo.